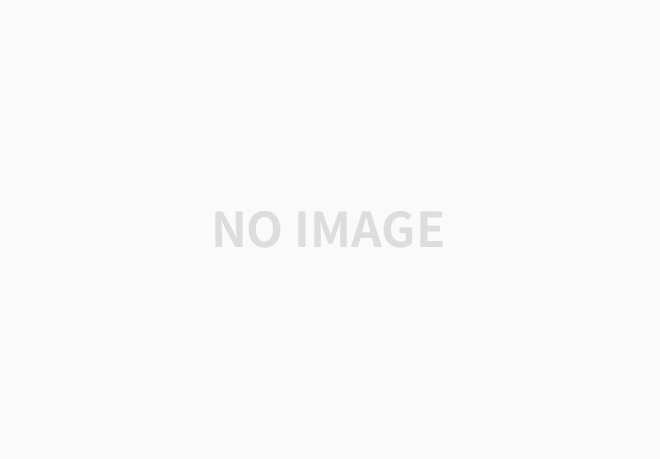
1. 정수 a,b 문자열 변환 (sprintf )
2. a+b, b+a 정의 (strcpy, strcat 함수 )
3. a+b, b+a를 다시 정수로 변환해서 대소 비교(atoi 함수)
#include <stdio.h>
#include <stdbool.h>
#include <stdlib.h>
#include <string.h>
int solution(int a, int b) {
int answer = 0;
char stra[10], strb[10];
sprintf(stra,"%d",a);
sprintf(strb,"%d",b);
char* sa = (char*)malloc(sizeof(char)*10);
char* sb = (char*)malloc(sizeof(char)*10);
strcpy(sa, &stra);
strcat(sa, strb);
strcpy(sb, &strb);
strcat(sb, stra);
int ia = atoi(sa);
int ib = atoi(sb);
if((a<1) || (b>10000))
return -1;
if(ia >= ib)
return ia;
else
return ib;
return answer;
}
1) sprintf
- 문자열 사이에 서식문자를 이용해 재조합하여 새로운 문자열을 만듬
- 출력하는 결과 값을 변수에 저장하게 해주는 기능 (string=printf -> sprintf)
- 기술된 형식(format)의 문자열로 변환
#include <stdio.h>
int sprintf(char *buffer, const char *format-string, arument-list);
입력 매개변수
- char *buffer : 출력값을 저장할 문자열
- const char *format : 서식 문자열
- ... 가변 인자 리스트
반환값
- 버퍼에 출력한 문자 개수
sprintf(문자열, "%d", 정수); //정수를 10진 형태의 문자열로 반환
sprintf(문자열, "%x", 정수); //정수를 16진 형태의 문자열로 반환
sprintf(문자열, "%X", 정수); //정수를 16진 대문자 형태의 문자열로 반환
#include <stdio.h>
int main()
{
char num1[10];
char num2[10];
int n1;
int n2;
scanf("%d %d", &n1, &n2);
sprintf(num1, "%d", n1);
sprintf(num2, "%d", n2);
printf("%s %s", num1, num2);
}
2) strcpy(dst, src)
- 문자열을 복사하는 함수
- dst와 src는 모두 문자 배열로 src를 dst로 복사
#include <stdio.h>
#include <string.h>
int main(void)
{
char src[] = "hello";
char dst[6];
strcpy(dst, src);
printf("복사된 문자열=%s\n", dst);
return 0;
}
3) strcat
- 문자열 뒤에 다른 문자열 연결
#include <stdio.h>
#include <string.h>
int main(void)
{
char s[11] = "hello";
strcat(s, "world");
printf("%s \n", s);
return 0;
}
4) 문자열 수치로 변환
- 문자열을 정수값으로 변환하려면 atoi()를 사용하거나 sscanf()를 사용
#include <stdio.h>
#include <string.h>
int main(void)
{
const char s[] = "100";
char t[100] = "";
int i;
printf("%d\n",atoi("100")); //atoi()는 문자열 "100"을 정수 100으로 바꿈
sscanf(s, "%d", &i) ; //문자열 "100"을 정수 100으로 i에 저장
sprintf(t, "%d",100); //정수 100을 문자열 "100"으로 t에 저장
return 0;
}
'C언어 > 프로그래머스' 카테고리의 다른 글
[c언어] 두 수의 연산값 비교하기 (0) | 2023.08.28 |
---|---|
[c언어] 문자열 곱하기 (0) | 2023.08.27 |
[c언어] 문자 리스트를 문자열로 변환하기 (1) | 2023.08.27 |
[c언어] 문자열 섞기 (0) | 2023.08.27 |
[c언어] 문자열 겹쳐쓰기 (0) | 2023.08.27 |